DATA SCIENCE INTERVIEW QUESTIONS PART 1
Interview Questions
1. INTRODUCTION TO DATA SCIENCE
- What is a Data Science?
Who is a Data Scientist?
Who can become a Data Scientist?
What is an Artificial Intelligence?
What is a Machine Learning?
What is Deep Learning?
Artificial Intelligence vs. Machine Learning vs. deep Learning
Real-Time Process of Data Science
Data Science Real-Time Applications
Technologies Used in Data Science
Prerequisites Knowledge to Learn Data Science
2. INTRODUCTION TO MACHINE LEARNING
- What is Machine Learning?
Machine Learning Vs Statistics
Traditional Programming Vs Machine Learning
How Machines Will Learn Like Human Learning
Machine Learning Engineer Responsibilities
Types of Machine Learning
Supervised learning
Un-Supervised learning
Reinforcement Learning
3.CORE PYTHON PROGRAMMING
- PYTHON Programming Introduction
History of Python
Python is Derived from?
Python Features
Python Applications
Why Python is Becoming Popular Now a Day?
Existing Programming Vs Python Programming
Writing Programs in Python Top Companies Using Python
Python Programming Modes
o Interactive Mode Programming
o Scripting Mode Programming
Flavors in Python, Python Versions
Download & Install the Python in Windows & Linux
How to set Python Environment in the System?
Anaconda – Data Science Distributor
Downloading and Installing Anaconda, Jupyter Notebook &
Spyder
Python IDE – Jupyter Notebook Environment
Python IDE – Spyder Environment
Python Identifiers(Literals), Reserved Keywords
Variables, Comments
Lines and Indentations, Quotations
Assigning Values to Variables
Data Types in Python
Mutable Vs Immutable
Fundamental Data Types: int, float, complex, bool, str
Number Data Types: Decimal, Binary, Octal, Hexa Decimal &
Number Conversions
Inbuilt Functions in Python
Data Type Conversions
Priorities of Data Types in Python
Python Operators - o Arithmetic Operators
o Comparison (Relational) Operators
o Assignment Operators
o Logical Operators
o Bitwise Operators
o Membership Operators
o Identity Operators - Slicing & Indexing
o Forward Direction Slicing with +ve Step
o Backward Direction Slicing with -ve Step
Decision-Making Statements
o if Statement
o if-else Statement
o Elif Statement
Looping Statements
o Why do we use Loops in Python?
o Advantages of Loops
o for Loop - o Nested for Loop
o Using else Statement with for Loop
o while Loop
o Infinite while Loop
o Using else with Python while Loop
Conditional Statements
o break Statement
o continue Statement
o Pass Statement
4. ADVANCED PYTHON PROGRAMMING
- Advanced Data Types: List, Tuple, Set, Frozenset, Dictionary,
Range, Bytes & Bytearray, None
List Data Structure
o List indexing and splitting
o Updating List values
o List Operations
o Iterating a List
o Adding Elements to the List
o Removing Elements from the List
o List Built-in Functions
o List Built-in Methods
Tuple Data Structure
o Tuple Indexing and Splitting
o Tuple Operations
o Tuple Inbuilt Functions
o Where use Tuple
o List Vs Tuple
o Nesting List and Tuple
Set Data Structure
o Creating a Set
o Set Operations
o Adding Items to the Set
o Removing Items from the Set
o Difference Between discard() and remove()
o Union of Two Sets
o Intersection of Two Sets
o Difference of Two Sets
o Set Comparisons
Frozenset Data Structure
Dictionary Data Structure
o Creating the Dictionary
o Accessing the Dictionary Values o Updating Dictionary Values
o Deleting Elements Using del Keyword
o Iterating Dictionary
o Properties of Dictionary Keys
o Built-in Dictionary Functions
o Built-in Dictionary Methods
List Vs Tuple Vs Set Vs Frozenset Vs Dict
Range, Bytes, By tearray & None
Python Functions
o Advantage of Functions in Python
o Creating a Function
o Function Calling
o Parameters in Function
o Call by Reference in Python
o Types of Arguments
Required Arguments
Keyword Arguments
Default Arguments
Variable-Length Arguments
Scope of Variables
Python Built-in Functions
Python Lambda Functions
String with Functions
o Strings Indexing and Splitting
o String Operators
o Python Formatting Operator
o Built-in String Functions
Python File Handling
o Opening a File
o Reading the File
o Read Lines of the File
o Looping through the File
o Writing the File
o Creating a New File
o Using with Statement with Files
o File Pointer Positions
o Modifying File Pointer Position
o Renaming the File & Removing the File
o Writing Python Output to the Files
o File-Related Methods
Python Exceptions
o Common Exceptions
o Problem without Handling Exceptions o except Statement with no Exception
o Declaring Multiple Exceptions
o Finally Block
o Raising Exceptions
o Custom Exception
Python Packages
o Python Libraries
o Python Modules
Collection Module
Math Module
OS Module
Random Module
Statistics Module
Sys Module
Date & Time Module
o Loading the Module in our Python Code
import Statement
from-import Statement
o Renaming a Module
Regular Expressions
Command Line Arguments
Object Oriented Programming (OOP)
o Object-oriented vs Procedure-oriented Programming
languages
o Object
o Class
o Method
o Inheritance
o Polymorphism
o Data Abstraction
o Encapsulation
Python Class and Objects
o Creating Classes in Python
o Creating an Instance of the Class
Python Constructor
o Creating the Constructor in Python
o Parameterized Constructor
o Non-Parameterized Constructor
o In-built Class Functions
o In-built Class Attributes
Python Inheritance
o Python Multi-Level Inheritance
o Python Multiple Inheritance o Method Overriding
o Data Abstraction in Python
Graphical User Interface (GUI) Programming
Python Tkinter
o Tkinter Geometry
pack() Method
grid() Method
place() Method
o Tkinter Widgets
5. DATA ANALYSIS WITH PYTHON NUMPY
- NumPy Introduction
o What is NumPy
o The Need for NumPy
NumPy Environment Setup
N-Dimensional Array (ndarray)
o Creating a Ndarray Object
o Finding the Dimensions of the Array
o Finding the Size of Each Array Element
o Finding the Data Type of Each Array Item
o Finding the Shape and Size of the Array
o Reshaping the Array Objects
o Slicing in the Array
o Finding the Maximum, Minimum, and Sum of the Array
Elements
o NumPy Array Axis
o Finding Square Root and Standard Deviation
o Arithmetic Operations on the Array
o Array Concatenation
NumPy Datatypes
o NumPy D type
o Creating a Structured Data Type
Numpy Array Creation
o Numpy. empty
o Numpy. Zeros
o NumPy.ones
Numpy Array from Existing Data
o Numpy. as array
NumPy Arrays within the Numerical Range
o Numpy. arrange
o NumPy. space
o Numpy. logspace
NumPy Broadcasting o Broadcasting Rules
NumPy Array Iteration
o Order of Iteration
F-Style Order
C-Style Order
o Array Values Modification
NumPy String Functions
NumPy Mathematical Functions
o Trigonometric Functions
o Rounding Functions
NumPy Statistical functions
o Finding the Min and Max Elements from the Array
o Calculating Median, Mean, and Average of Array Items
NumPy Sorting and Searching
NumPy Copies and Views
NumPy Matrix Library
NumPy Linear Algebra
NumPy Matrix Multiplication in Python
6. DATA ANALYSIS WITH PYTHON PANDAS
- Pandas Introduction & Pandas Environment Setup
o Key Features of Pandas
o Benefits of Pandas
o Python Pandas Data Structure
Series
Data Frame
Panel
Pandas Series
o Creating a Series
Create an Empty Series
Create a Series using Inputs
o Accessing Data from Series with Position
o Series Object Attributes
o Retrieving Index Array and Data Array of a Series Object
o Retrieving Types (type) and Size of Type (item size)
o Retrieving Shape
o Retrieving Dimension, Size, and Number of Bytes
o Checking Emptiness and Presence of NaNs
o Series Functions
Pandas Data Frame
o Create a Data Frame
Create an Empty Data Frame
Create a data frame using Inputs Column Selection, Addition & Deletion
Row Selection, Addition & Deletion
Data Frame Functions
Merging, Joining & Combining Data Frames
Pandas Concatenation
Pandas Time Series
o Datetime
o Time Offset
o Periods
o Convert String to Date
Viewing/Inspecting Data (loc & loc)
Data Cleaning
Filter, Sort, and Group by
Statistics on Data Frame
Pandas Vs NumPy
Data Frame Plotting
o Line: Line Plot (Default)
o Bar: Vertical Bar Plot
o Barh: Horizontal Bar Plot
o Hist: Histogram Plot
o Box: Box Plot
o Pie: Pie Chart
o Scatter: Scatter Plot
7. DBMS – Structured Query Language
- Introduction & Models of DBMS
SQL & Sub Language of SQL
Data Definition Language (DDL)
Data Manipulation Language (DML)
Data Query/Retrieval Language (DQL/DRL)
Transaction Control Language (TCL)
Data Control Language (DCL)
Installation of MySQL & Database Normalization
Sub Queries & Key Constraints
Aggregative Functions, Clauses & Views
8. Importing & Exporting Data
- Data Extraction from CSV (pd.read_csv)
Data Extraction from TEXT File (pd.read_table)
Data Extraction from CLIPBOARD (pd.read_clipboard)
Data Extraction from EXCEL (pd.read_excel)
Data Extraction from URL (pd.read_html)
Writing into CSV (df.to_cs Writing into EXCEL (df.to_excel)
Data Extraction from DATABASES
o Python MySQL Database Connection
Import my sql. connector Module
Create the Connection Object
Create the Cursor Object
Execute the Query
9.DATA VISUALIZATION WITH PYTHON MATPLOTLIB
- Data Visualization Introduction
Tasks of Data Visualization
Benefit of Data Visualization
Plots for Data Visualization
Matplotlib Architecture
General Concept of Matplotlib
MatPlotLib Environment Setup
Verify the matplotlib Installation
Working with PyPlot
Formatting the Style of the Plot
Plotting with Categorical Variables
Multi-Plots with Subplot Function
Line Graph
Bar Graph
Histogram
Scatter Plot
Pie Plot
3Dimensional – 3D Graph Plot
mpl_toolkits
Functions of Mat Plot Lib
Contour Plot, Quiver Plot, Violin Plot
3D Contour Plot
3D Wireframe Plot
3D Surface Plot
Box Plot
o What is a Boxplot?
o Mean, Median, Quartiles, Outliers
o Inter Quartile Range (IQR), Whiskers
o Data Distribution Analysis
o Boxplot on a Normal Distribution
o Probability Density Function
o 68–95–99.7 Rule (Empirical rule)
10.DATA VISUALIZATION
11. Machine Learning
12. Supervised Machine Learning
13. Unsupervised Machine Learning
14. Data Preprocessing in Machine Learning
15. Classification Algorithms in Machine Learning
|
Upskill & Reskill For Your Future With Our Software Courses
Data Analyst Course in Hyderabad
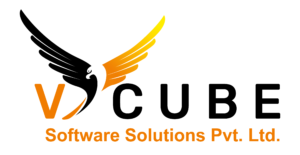
Quick Links
- Home
- About Us
- Courses
- Contact Us
Other Pages
Contact Info
- 2nd Floor Above Raymond’s Clothing Store KPHB, Phase-1, Kukatpally, Hyderabad
- +91 7675070124, +91 9059456742
- contact@vcubegroup.com